In our previous post Understanding Blazor’s Pre-rendering Behavior, we explored how Blazor’s default pre-rendering behavior can lead to unexpected issues when injecting services, particularly in hybrid (server + WASM) projects. One workaround is to register services on both the server and client, but this can lead to your components rendering twice — once on the server and again on the client during hydration.
To avoid re-running logic and unnecessary performance hits, Blazor provides a mechanism to persist state between the server prerendering and client hydration phases. In this article, we’ll demonstrate the problem and then walk through how to use PersistentComponentState
to solve it.
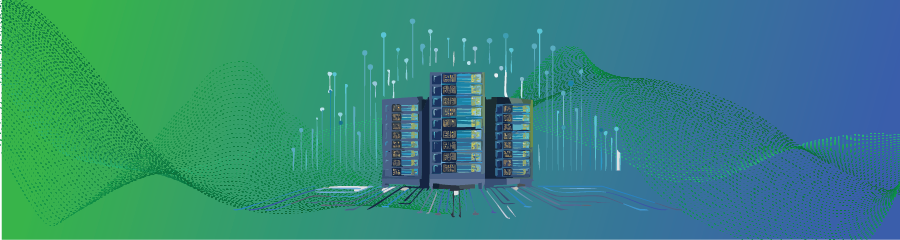
Understanding Blazor’s Pre-rendering Behavior: Why Your Service Injection Might Fail
If you’ve been working with Blazor lately, especially using the latest project templates in .NET 8, you might have run into a frustrating and seemingly inexplicable error when injecting services into your components:
InvalidOperationException: Cannot provide a value for property 'Service' on type MyPage. There is no registered service of type MyService.
You double-check your Program.cs
, and your service is clearly registered:
builder.Services.AddScoped<MyService>();
So what’s going on?
Read more...

Implementing a Mock-Driven Approach in Blazor Applications
When starting a project, you typically work with clients on UI sketches. The client provides a list of requirements or pages they want to see. You begin building the client app and backend, designing the database schema, etc. After a few weeks, you present the first demo to the client, only to discover they want to change everything.
Read more...

A Practical Guide to ASP.NET Applications: Designing Robust CRUD WebAPIs (Part2)
This article offers an approach to designing, implementing, and unit-testing CRUD APIs (Create, Read, Update, Delete). We will explore best practices for designing web APIs, discuss how to implement pagination, searching, and outline methods for organizing models effectively.
Read more...

A Practical Guide to ASP.NET Applications: The Structure (Part 1)
In this article, we will discuss structuring an ASP.NET Core project to prepare it for large-scale commercial software solutions. Topics covered include project structure as well as service boundaries and responsibilities. To see a practical example of the concepts discussed, please check out the DevCoreApp repository.
Read more...Introducing the LogScope: the cleaner way of logging and tracing methods.
LogScope is not yet another logger. It is an extension of existing logging libraries that provides alternative way of logging and measuring a performance of critical parts of code. It also helps building a cleaner picture of executing asynchronous calls. It can used along with any existing logging provider.
The whole idea is based on a logging scope. Based on IDisposable
interface, scope:
- Logs it instantiation and disposal;
- Can log lines as part of the scope;
- Can be created from logging manager or as part of another scope (a nested scope);